This article is about Programming
How to build an array using C++
By NIIT Editorial
Published on 14/07/2021
8 minutes
An array is a collection of elements with the same data type that are arranged in a logical order. In memory, they are organized in sequential order. A data structure that holds elements of the same type is known as an array. In C++, unlike in Java, array elements are not recognized as objects. Arrays in C++ will be discussed in this article.
Arrays In C++
Imagine you are at a library and I tell you to arrange all the books under the label XYZ genre at one place one above the other. As we discussed this is an array, a collection of records that are arranged in a logical order. The data type in our case is XYZ genre, and all of the books you collected have the same publishers. A common name is used to address all of the elements in an array. Let us start by understanding what single-dimensional arrays are:
Array with a single dimension
A Single Dimensional Array can be declared using the following syntax:
We have a data type that can be int, float, or double, and it can be any of the basic data types. The array's name is Array Name, and the array's size is declared. The array in our previous example will be,

Let’s consider another example:

The array arr will have a size of 50 and will contain elements of type int.
Arrays In C++: Array Size
When an array is declared, the size of the array is specified. The array's size cannot be modified once it has been specified. The array is then given that much memory space by the compiler.
Consider the Example

We have an array arr of type int in the previous example. The array size has been set to 50. This indicates that the array will have 50 consecutive memory locations to work with.
Array Index And Initialization
The array index is the number that is associated with each location in an array. It goes from 0 through the last element, which is the array's size minus one. We start counting from zero, not one, thus the minus one is there. An array's indices always begin at zero. Consider this example, this is the marks array.

The values in this array are 82,56,47,93,78, and the indices are 0,1,2,3,4,5. If we wish to represent an element at index 4, we use marks [4], which will display the number 78.
The array contains all zero values by default. The array is initialised at the moment of declaration. If the user enters the array value as and when needed, this can also be done later.
Let's have a look at how initialization works during the declaration process.
Initialization During Declaration
An array can be initialized as it is being declared. This is accomplished by providing the array elements during the declaration process. The array size is also fixed in this case, and it is determined by us.
Consider the code,
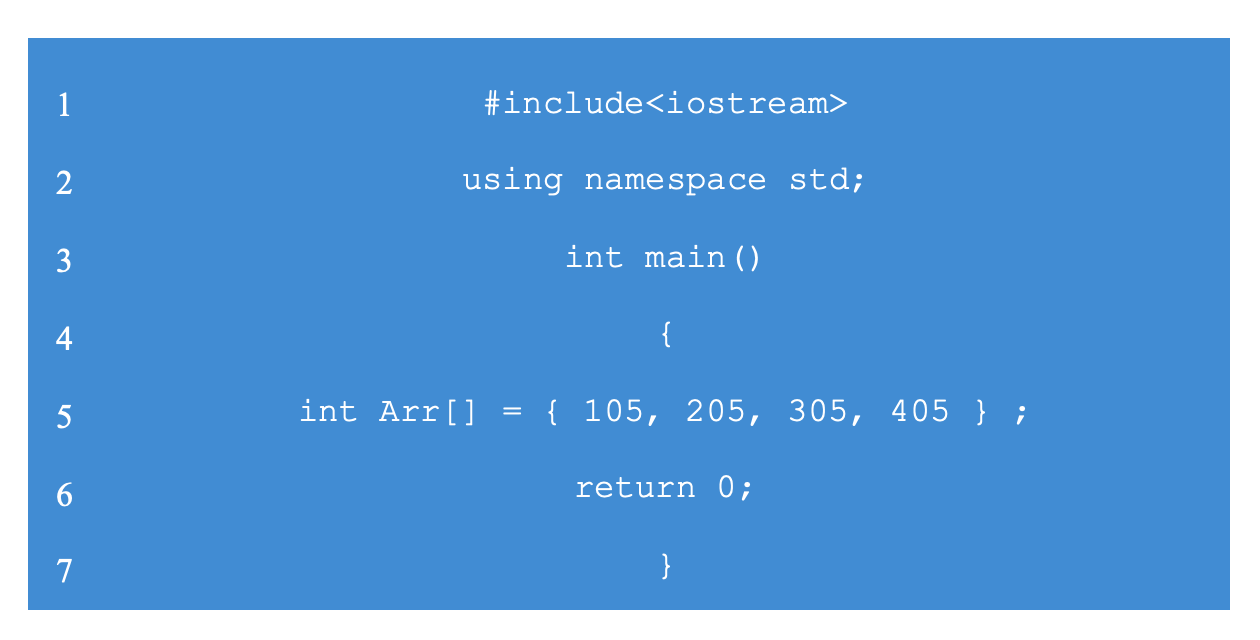
Explanation
We create an array of type int with the name Arr in the preceding example. The array elements are specified directly. Counting the number of entries in our array determines the array's size. The size is 4 in this example.
In the next section of this article on C++ arrays, we'll look at how user-initiated arrays function.
Initialization By A User
The size of the array is determined by the user in this technique. In this situation, we'll need a variable to store the array's size and a for-loop to receive the array's entries. At the time of declaration, we assign a random size and only use it as needed. The initial size is usually on the larger side. The for-loop is controlled by the variable j.
Consider the example,
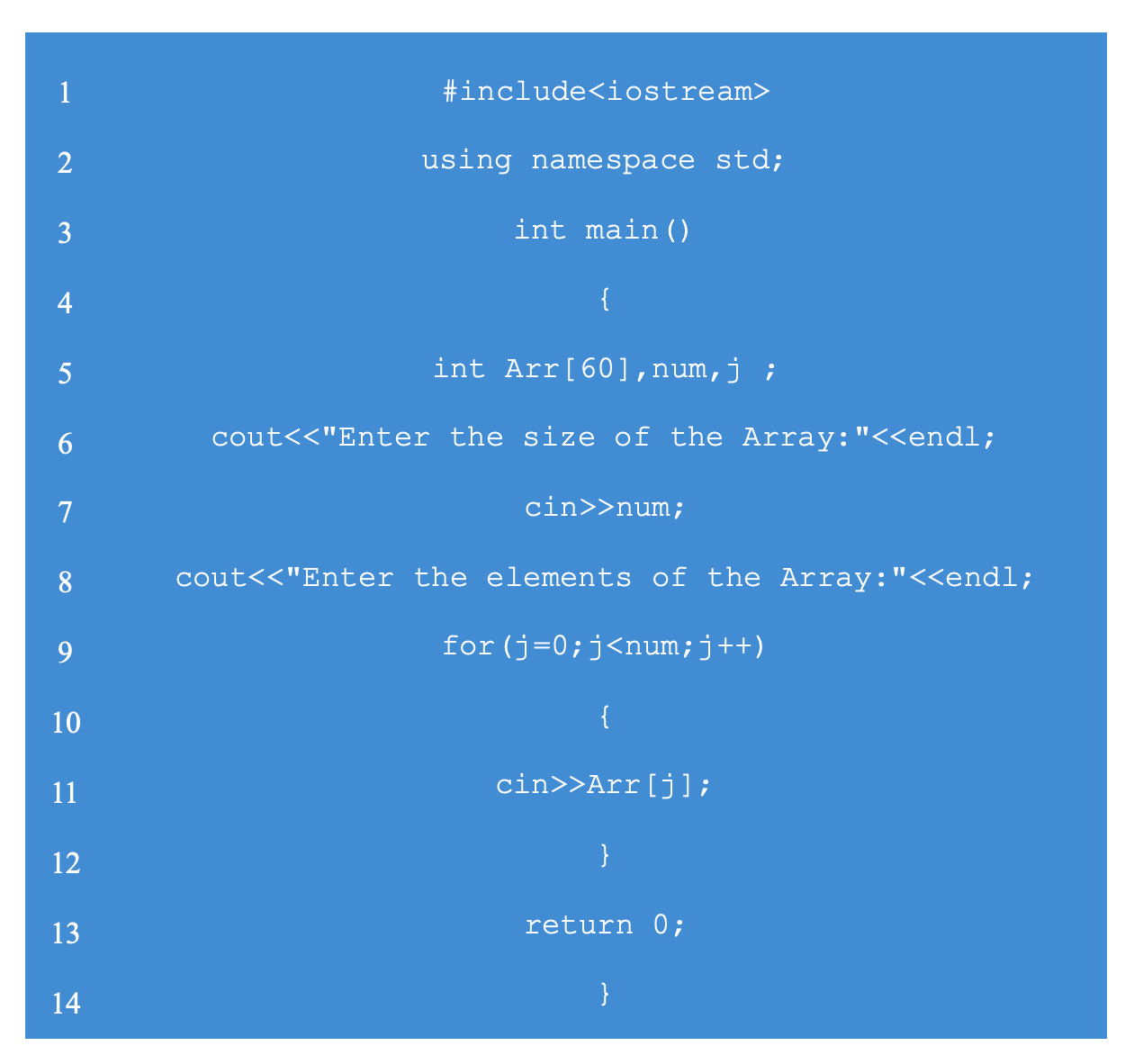
Output
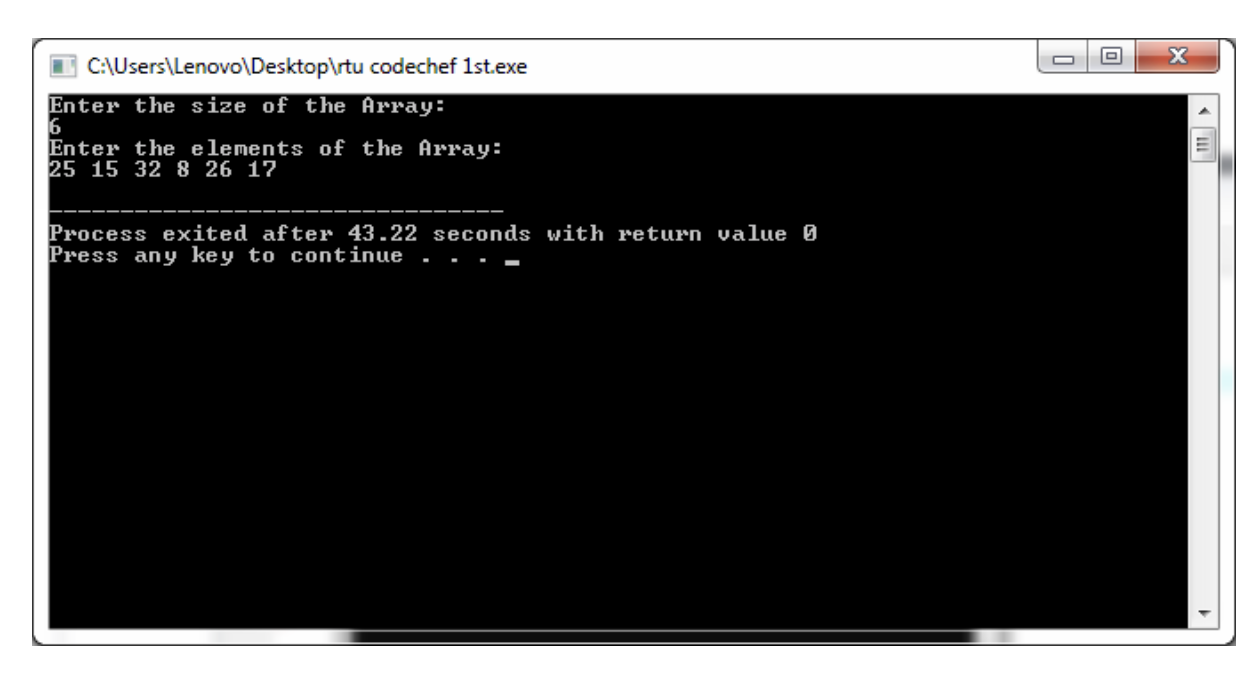
Explanation
In the above programme, we declare an array of size 60. The user is then asked to specify the amount of entries he wants to include in this array. The user's array elements are then accepted.
Arrays In C++: Displaying the Array
The for-loop is also required for displaying the array. We go through the entire array and display the elements. Here is an example,
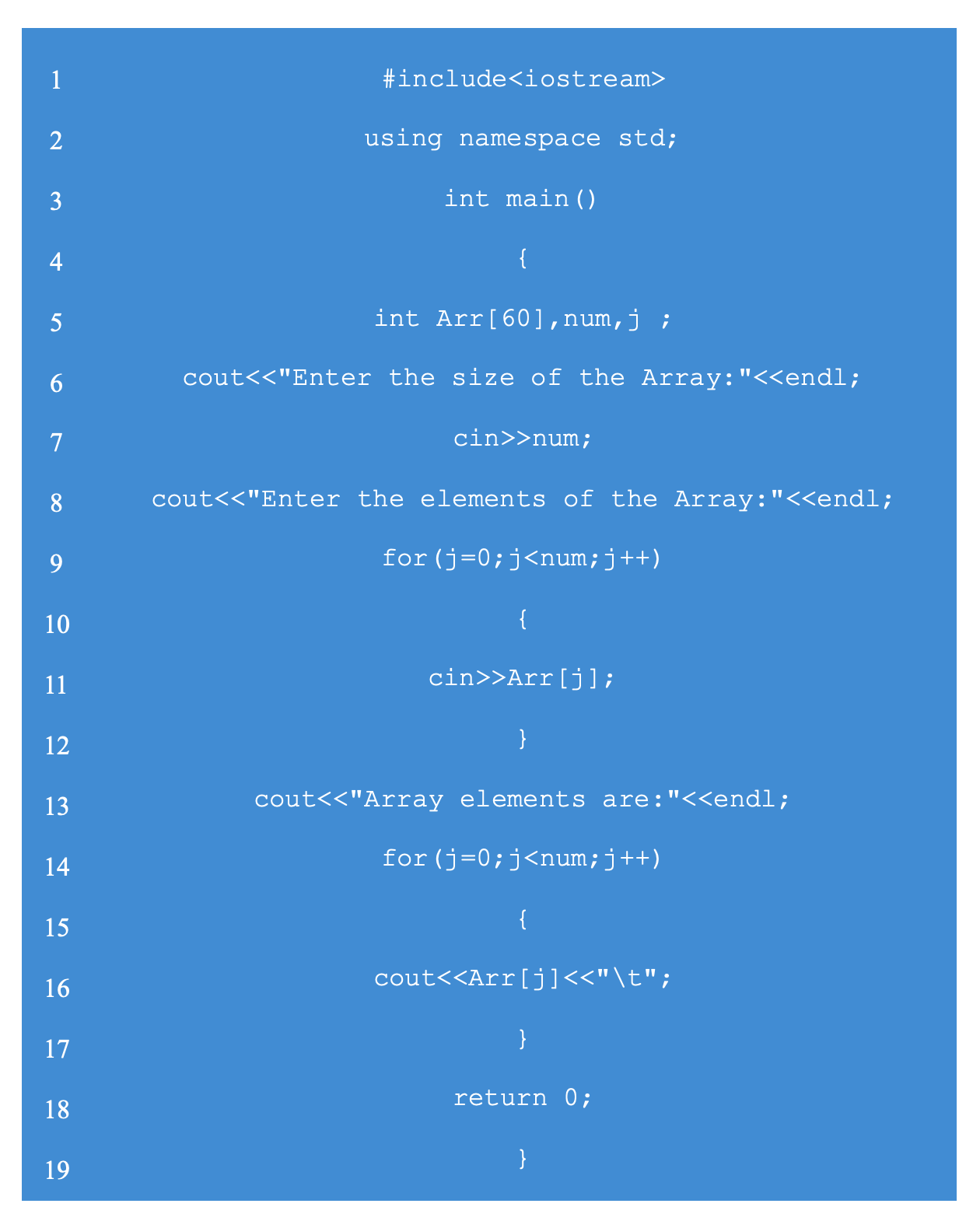
Output
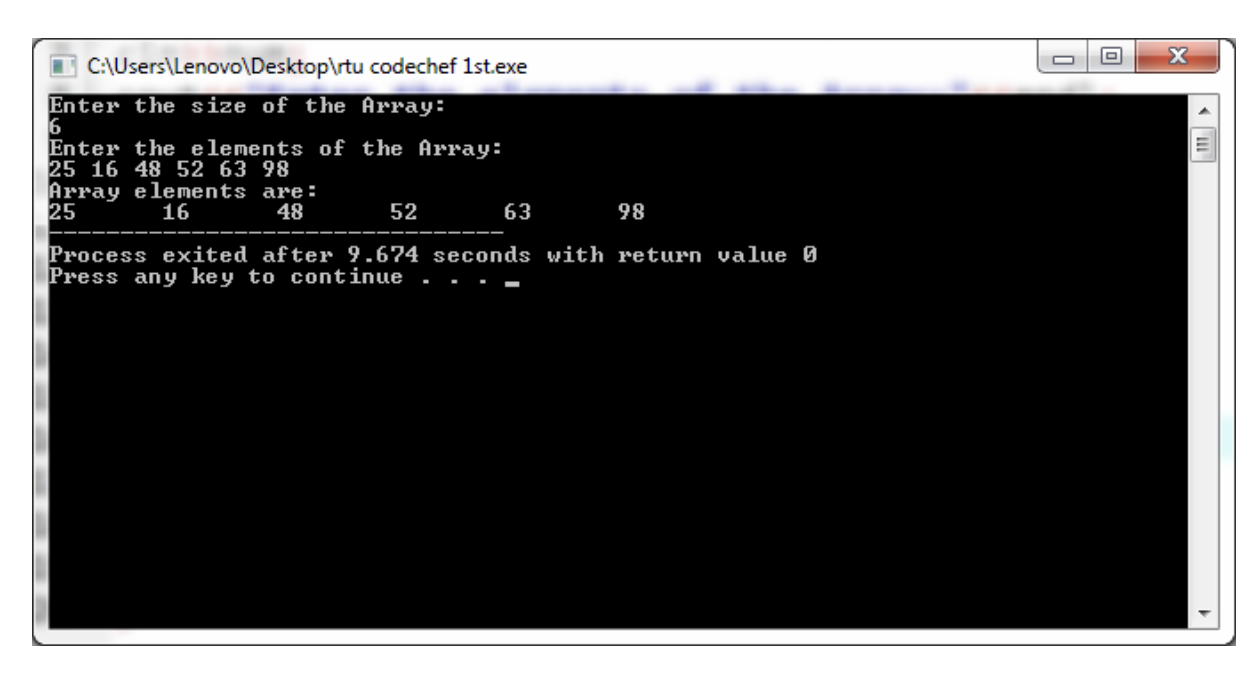
Explanation
We declare an array of size 60. The user is then asked to specify the amount of elements he wants in this array. The array elements submitted by the user are then accepted. The array elements are then displayed using a for-loop once more.
Moving on with these arrays in C++ article,
Accessing Array At Any Point
Using the array index to access array elements is a simple process. Take a look at the code in the next section.

Output

Explanation
We have an array of size 6 in the programme above. Using array index, we enter elements in various locations. To get the aforementioned output, we print the array.
All array elements are zero by default.
What happens if the array size is exceeded?
If we try to access elements that are out of bounds in C++, the compiler may not display an error, but we will not get valid output.
This brings us to the final bit of this array in C++ article,
Multi Dimensional Array
Multi-dimensional arrays are arrays of arrays. This is due to the fact that each element in a multi-dimensional array has its own array. For loops to traverse through a multidimensional array, we need n, which varies according to the dimensions.
Declaring Multi Dimensional Arrays Syntax
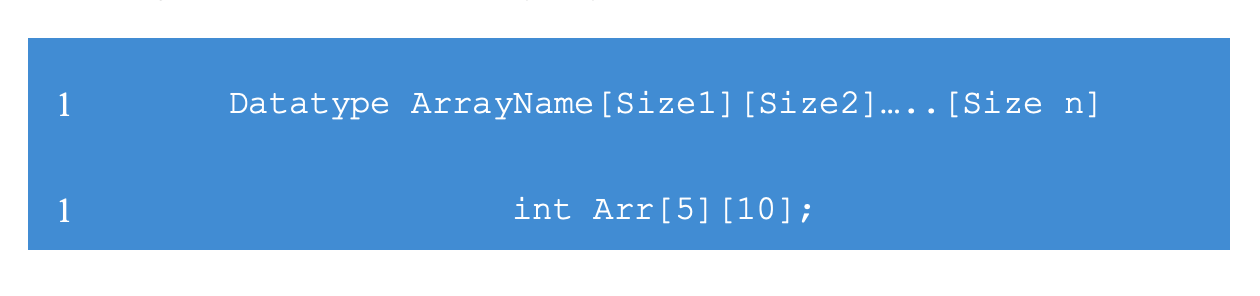
Consider the example,
The above array will have a size of 5*10, or 50 elements. Similarly, two, three, or even more dimensional arrays are possible. One for-loop is required for each dimension. As a result, the two-dimensional array necessitates two, while the three-dimensional array necessitates three.
Consider the code

Output
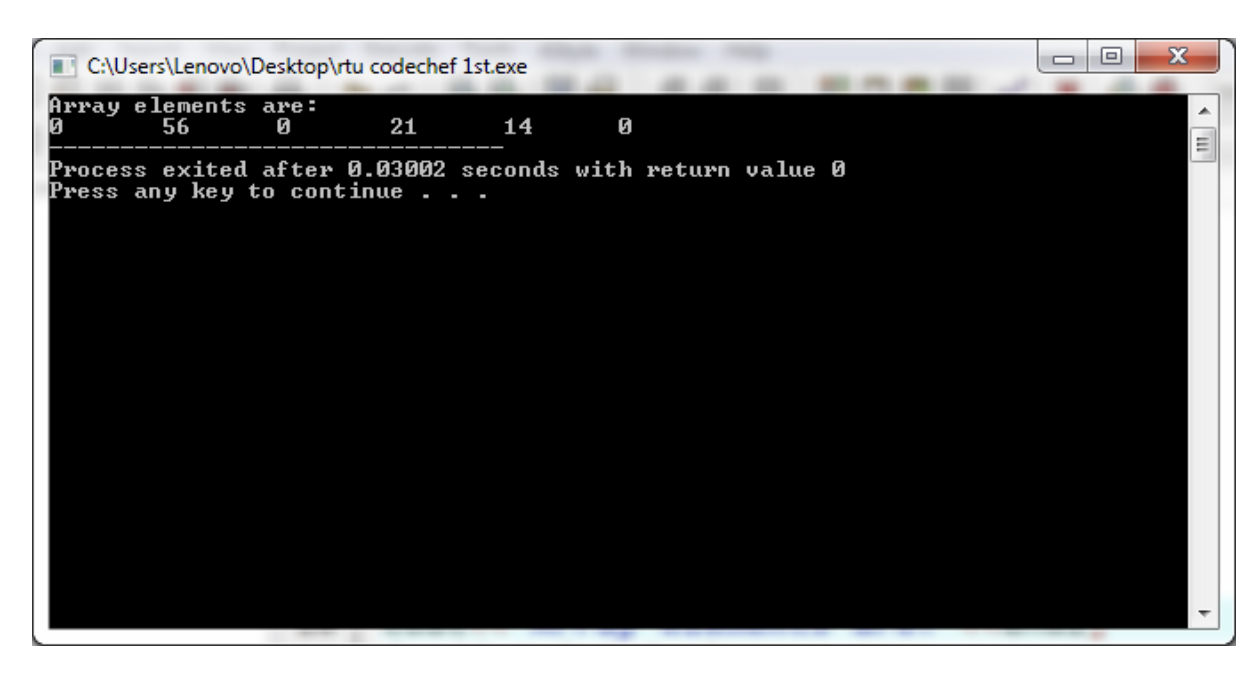
Explanation
A 2*3 matrix is displayed in the code above. There are two rows and three columns in this array. For-loops, we have two. Each is in charge of one of the array's dimensions. Rows are taken care of by the outer for-loop, while columns are taken care of by the inner for-loop.
Similarly, we may construct code for a three-dimensional array, with three for-loops and one for-loop controlling each dimension.
This article on ‘Arrays in C++' has now come to a close. If you want to learn more, courses offered by NIIT can help you in that.
PGP in Full Stack Product Engineering
Become an Industry-Ready StackRoute Certified Full Stack Product Engineer who can take up Product Development and Digital Transformation projects. Job Assured Program* with a minimum CTC of ₹7LPA*
Job Assured Program*
Easy Financing Options