We're working on curating more amazing courses for you! Until then, why not explore other categories or let us know what you're interested in? Your next learning adventure awaits!.

Try our course navigator
Book a visit at our learner hub
Explore tailored pathways and unlock your potential with expert guidance.
Delhi
Nehru Place
Mumbai
Bandra Hill Road
Bengaluru
Richmond Road
The NIIT advantage
Learners around the world
Hiring partners hire from us
Countries, one mission
Learner success stories
My journey at NIIT has been a transformative experience filled with learning & growth that have significantly shaped my professional & personal skills. With time, I adapted to the new learnin...
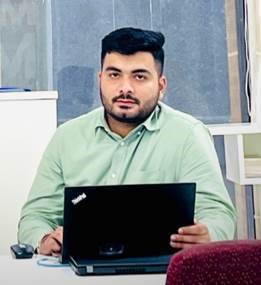
Rahul Poonia
"I'm grateful to NIIT - StackRoute for their incredible support. The course was challenging, but teachers were always available"...

Siddhesh Satish Kulkarni
Hi, I’m Vishal Singh. I graduated in year 2021 after my Hospitality Management I joined NIIT Full Stack Digital marketing Program. Under that program I learnt so many technical Skills, be it from SEO,...
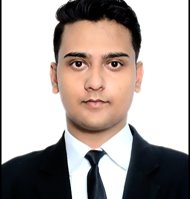
Vishal Singh
HiveMinds
"Choosing the gNIIT program was the best decision—I turned my passion into a profession"...

Ruchika
NIIT was really good, for me at the time of training I learnt many things and mock interview really helped to crack the interview. The classes were very good and our faculty is very supportive, overal...

Vikash Kumar
"gNIIT transformed me from a graduate into an IT professional!"...
